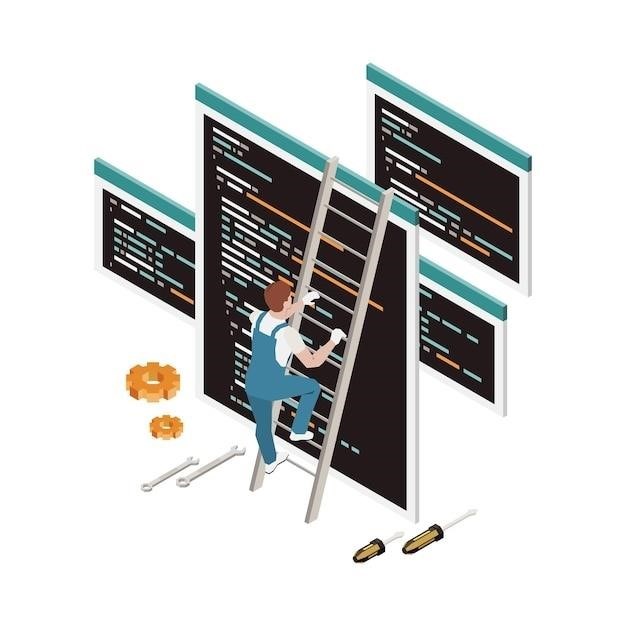
the complete linux coding manual
This comprehensive manual will guide you through the world of Linux programming, from the fundamentals of the kernel to advanced techniques for creating powerful applications. It provides a step-by-step introduction to the core concepts of Linux, including system calls, the C programming language, and the Linux kernel.
You will gain insights into the Linux Programming Interface, learn how to write kernel modules, and explore best practices for debugging and testing. This manual serves as a valuable resource for both beginners and experienced programmers who wish to master the art of Linux development.
Introduction
Welcome to the world of Linux programming, a realm where you can unlock the power of one of the most versatile and influential operating systems in history. This manual serves as your guide to navigating the complex yet rewarding landscape of Linux development.
Linux, renowned for its open-source nature, offers a platform for innovation and creativity. It empowers developers to explore the depths of the system, customize its behavior, and craft applications that seamlessly integrate with the operating system. Whether you’re a seasoned programmer seeking to expand your horizons or a curious newcomer eager to embark on a coding journey, this manual provides the essential foundation for success.
From understanding the fundamental principles of the Linux kernel to mastering the art of writing kernel modules, this manual will equip you with the knowledge and skills needed to become a proficient Linux programmer. Get ready to delve into the exciting world of Linux development and unlock the potential to create remarkable applications that shape the future of computing.
The Linux Kernel
The Linux kernel is the heart of the Linux operating system, acting as the intermediary between hardware and software. It manages the system’s resources, including memory, CPU, and peripherals, and provides a foundation for all other software to run. Understanding the Linux kernel is essential for any aspiring Linux programmer, as it unlocks the potential to optimize performance, enhance security, and customize the system’s behavior.
The kernel, written primarily in the C programming language, is a complex and intricate piece of software, designed to handle a multitude of tasks. It orchestrates the execution of processes, manages file systems, facilitates communication between different parts of the system, and ensures the smooth operation of the entire operating environment.
As you delve deeper into Linux programming, you’ll discover the power of the kernel and its role in shaping the user experience. From understanding how the kernel handles interrupts and system calls to exploring its internal structures and algorithms, this manual will guide you through the intricacies of the Linux kernel and empower you to create applications that interact with the system at its core.
Kernel Module Programming
Kernel modules are powerful extensions that allow you to customize and enhance the functionality of the Linux kernel without requiring a system reboot. These modules are dynamically loaded and unloaded, providing a flexible way to add new drivers, file systems, or other features to your system.
Kernel module programming requires a deep understanding of the kernel’s internal workings and a strong grasp of C programming; You’ll need to learn how to interact with kernel data structures, manage memory, and ensure your module integrates seamlessly with the existing kernel code.
This manual will guide you through the process of creating and managing kernel modules, providing practical examples and insightful explanations. You’ll discover how to write code that interacts with device drivers, implement new system calls, and extend the kernel’s capabilities to meet your specific needs.
The Linux Programming Interface
The Linux Programming Interface (LPI) is the cornerstone of Linux application development, providing a standardized set of tools and functions that allow you to interact with the kernel and manage system resources. It defines how applications communicate with the operating system, ensuring compatibility and portability across various Linux distributions.
The LPI encompasses a wide range of functionalities, including system calls, library functions, and header files. It provides a comprehensive framework for managing processes, files, network connections, and other system-level operations.
This manual will delve into the intricacies of the LPI, exploring its key components and demonstrating how to utilize them effectively. You’ll learn about the structure of system calls, the role of libraries like libc, and how to leverage the LPI to build robust and efficient Linux applications.
System Calls
System calls are the primary means by which user-space applications interact with the Linux kernel. They act as the bridge between the application and the operating system, enabling access to privileged kernel functions. System calls are invoked through the syscall
instruction, which transfers control to the kernel and executes the requested operation.
The LPI defines a rich set of system calls that cover a wide range of functionalities. Some common examples include⁚
open
⁚ Opens a file or device for reading, writing, or both.read
⁚ Reads data from a file or device.write
⁚ Writes data to a file or device.fork
⁚ Creates a new process that is a copy of the current process.exec
⁚ Loads and executes a new program in the current process.
Understanding system calls is crucial for Linux programmers as they provide the building blocks for many essential system operations. This manual will provide detailed explanations of key system calls, their usage, and the parameters involved.
The C Programming Language
C is the foundational language of the Linux operating system, serving as the primary language for both the kernel and many system utilities. Its low-level access to memory and hardware, combined with its efficiency and portability, makes it ideal for system programming. The C language offers a wide array of features that are essential for Linux development, including⁚
- Pointers⁚ Pointers allow direct manipulation of memory addresses, enabling efficient resource management and system-level interaction.
- Data Structures⁚ C provides flexible data structures like arrays, structs, and unions, which are essential for organizing and managing complex data.
- Function Pointers⁚ Function pointers allow for dynamic function calls, enhancing code flexibility and modularity.
- Standard Library⁚ The C standard library offers a rich set of functions for input/output, string manipulation, memory management, and other essential operations.
This manual will delve into the intricacies of the C language, focusing on its key concepts and their application within the Linux environment. You will learn how to write effective C code for Linux, understanding the nuances of memory management, system calls, and kernel programming.
Linux Distros for Programming
Choosing the right Linux distribution for programming can significantly impact your development experience. Each distro offers a unique blend of features, package management systems, and community support that caters to different programming preferences. Here are some popular Linux distros tailored for programmers⁚
- Ubuntu⁚ Known for its user-friendliness and extensive software repositories, Ubuntu provides a stable and reliable platform for various programming tasks.
- Fedora⁚ Fedora is a bleeding-edge distribution that prioritizes innovation and cutting-edge technologies, making it ideal for developers interested in experimenting with the latest tools.
- Debian⁚ Debian is a stable and well-established distribution with a strong focus on security and reliability, making it a suitable choice for mission-critical development environments.
- Arch Linux⁚ Arch Linux is a highly customizable distribution that empowers users to tailor their system precisely to their needs, making it a favorite among experienced Linux users and developers.
- Gentoo⁚ Gentoo is a highly customizable distribution that allows users to compile software from source, offering maximum control over system configuration and optimization.
This manual will explore the strengths and weaknesses of each distro, providing insights into their package management systems, development tools, and community support. You will learn how to select the most appropriate distro for your programming needs, based on your specific requirements and preferences.
Coding Style
Consistent coding style is crucial for writing clean, readable, and maintainable code. In the context of Linux programming, a well-defined coding style ensures that your code adheres to the established conventions and practices within the Linux community. This promotes collaboration, improves code readability, and simplifies the maintenance process.
The Linux kernel itself follows a specific coding style outlined in the “Kernel Coding Style” document. This document provides guidelines for indentation, naming conventions, commenting, and other aspects of code formatting. Adhering to this style ensures that your code integrates seamlessly with the kernel source code and aligns with the community’s standards.
Beyond the kernel, many other Linux projects and organizations have their own coding style guides. It is essential to familiarize yourself with the specific coding style guidelines of the project or organization you are working on. Consistency in coding style across your codebase and within a team fosters a collaborative and efficient development environment.
Best Practices
In the realm of Linux programming, adhering to best practices is paramount for crafting robust, efficient, and maintainable code. These practices serve as guiding principles that enhance code quality, reduce errors, and facilitate collaboration. One fundamental best practice is modularity. Breaking down your code into smaller, self-contained modules enhances readability, simplifies debugging, and promotes code reusability. Each module should perform a specific function, making it easier to understand and maintain.
Another crucial practice is to prioritize code clarity. Employ meaningful variable and function names, write concise and descriptive comments, and avoid overly complex logic. Clear code is easier to understand, debug, and modify, fostering a more efficient development process. Furthermore, embracing defensive programming techniques helps prevent unexpected errors and ensures your code handles potential issues gracefully. This involves anticipating potential errors and implementing robust error handling mechanisms to gracefully handle unforeseen situations.
In addition, regular code reviews are essential for maintaining high code quality. Having peers review your code helps identify potential issues, improve code clarity, and ensure adherence to established best practices. By following these best practices, you can create high-quality, reliable, and maintainable Linux code that meets the demands of the ever-evolving software landscape.
Debugging and Testing
In the intricate world of Linux programming, debugging and testing are essential practices for ensuring the reliability and functionality of your code. Debugging involves identifying and resolving errors in your program, while testing verifies that your code behaves as expected and meets the desired specifications. A systematic approach to debugging is crucial for pinpointing the root cause of errors. Start by analyzing error messages, examining code execution flow, and utilizing debugging tools like gdb to step through your code line by line.
Testing plays a vital role in validating the correctness and robustness of your code. It involves executing your program with various inputs and comparing the outputs to the expected results. Unit testing, a popular approach, focuses on testing individual functions or modules in isolation. Integration testing, on the other hand, evaluates the interaction between different modules. By implementing a comprehensive testing strategy, you can ensure that your code adheres to the desired functionality and performs reliably under various conditions. The combination of effective debugging and rigorous testing is paramount for delivering high-quality, bug-free Linux applications.
Troubleshooting
Troubleshooting is an inevitable part of the Linux programming journey. Encountering errors and unexpected behavior is common, and the ability to effectively diagnose and resolve these issues is crucial. When faced with a problem, start by gathering information. Examine error messages, logs, and system status to gain insights into the nature of the issue. Utilize debugging tools like gdb to step through your code and identify the source of the problem.
If the error is related to system configuration or dependencies, consult documentation, online forums, and community resources to find solutions. Remember to verify that all necessary packages and libraries are installed and configured correctly. Sometimes, a simple reboot or clearing of cache can resolve unexpected behavior. For complex issues, consider seeking assistance from experienced Linux programmers or online communities. By approaching troubleshooting with a systematic and methodical approach, you can effectively identify and resolve problems, ensuring the smooth operation of your Linux programs.
Resources and Further Reading
The world of Linux programming is vast and constantly evolving, offering a wealth of resources for continuous learning and exploration. To delve deeper into specific areas, consider consulting authoritative books like “The Linux Programming Interface” by Michael Kerrisk, which provides a comprehensive guide to the Linux and UNIX programming interfaces. For kernel module programming, “The Linux Kernel Module Programming Guide” is an invaluable resource, offering practical instructions and insights into the intricacies of kernel development.
Online forums and communities like Stack Overflow, Reddit’s r/linux, and the Linux Foundation’s website are excellent platforms for asking questions, seeking advice, and engaging with other Linux enthusiasts. The Linux Documentation Project (LDP) provides a wide range of documentation, tutorials, and articles, covering various aspects of Linux programming. By exploring these resources, you can enhance your understanding of Linux programming, stay informed about the latest advancements, and connect with a vibrant community of fellow programmers.